728x90
반응형
환경변수 재설정
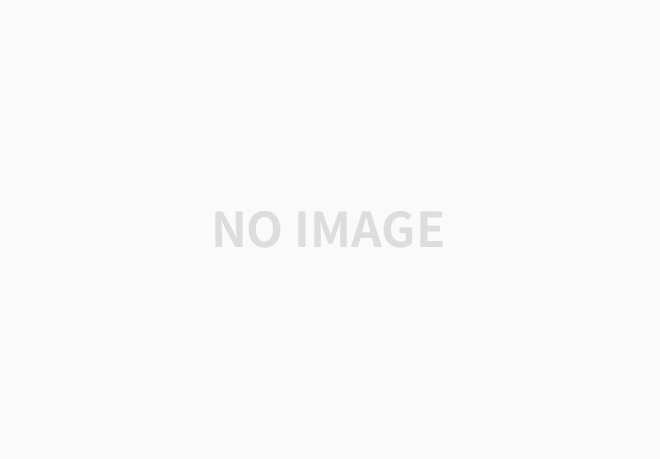
다시 프로젝트 쪽을 진행해보도록 하자.
6.7.2 버전에서 실행했기에 해당 버전을 archive에서 다운받고
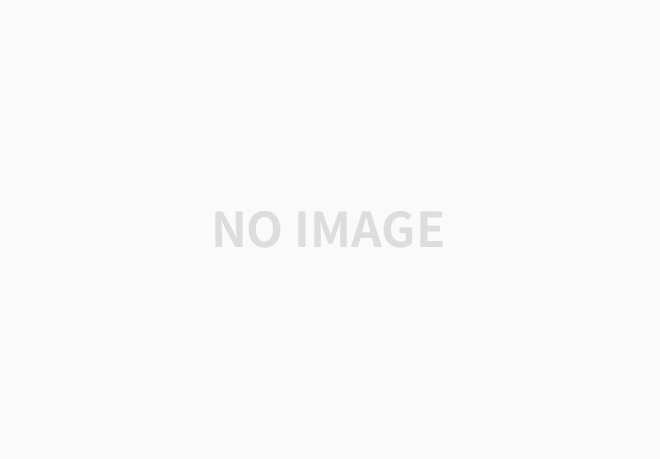
실행하는데 왜 dll 에러가 뜨지?
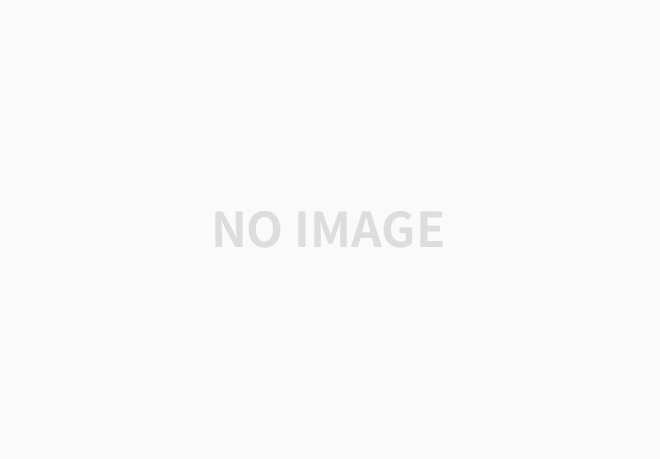
보아하니 환경변수 떄문인 것 같다.
C드라이브가 아니라 지금은 D 드라이브에 깔려 있다.
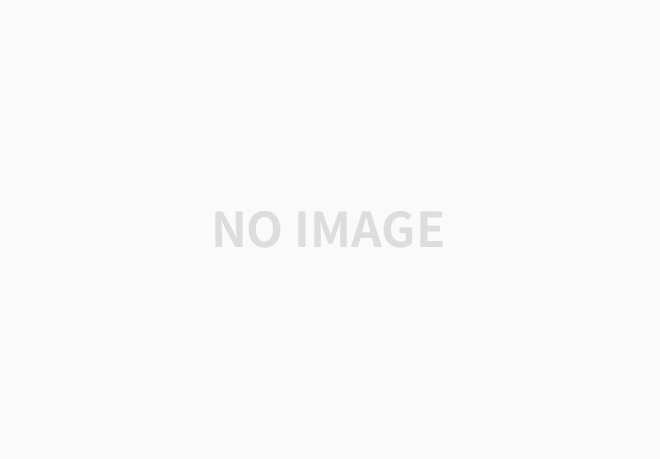
환경 변수를 설정하니 이제서야 됐다.
#include <iostream>
#include <vector>
#include <QtOpenGL/QtOpenGL>
#include <QtOpenGLWidgets/QtOpenGLWidgets>
#include <QMouseEvent>
#include <QColorDialog>
#include <QVBoxLayout>
#include <QPushButton>
using namespace std;
class Scene : public QOpenGLWidget, protected QOpenGLFunctions {
vector<pair<QColor, vector<QPointF>>> lines; // 선들과 각 선의 색상
vector<QPointF> currentLine; // 현재 그리고 있는 선
QColor currentColor = Qt::black; // 기본 색상은 검정
public:
Scene(QWidget* parent = nullptr) : QOpenGLWidget(parent) {}
void setColor(const QColor& color) {
currentColor = color;
update();
}
protected:
void initializeGL() override {
initializeOpenGLFunctions();
glClearColor(1.0f, 1.0f, 1.0f, 1.0f); // 흰색 배경
}
void paintGL() override {
glClear(GL_COLOR_BUFFER_BIT);
// 저장된 모든 선을 그린다
for (const auto& line : lines) {
glColor3f(line.first.redF(), line.first.greenF(), line.first.blueF()); // 저장된 색상으로 선 그리기
glBegin(GL_LINE_STRIP);
for (const auto& point : line.second) {
glVertex2f(point.x(), point.y());
}
glEnd();
}
// 현재 그리고 있는 선을 그린다
glColor3f(currentColor.redF(), currentColor.greenF(), currentColor.blueF());
glBegin(GL_LINE_STRIP);
for (const auto& point : currentLine) {
glVertex2f(point.x(), point.y());
}
glEnd();
glFlush();
}
void resizeGL(int w, int h) override {
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(0, w, h, 0, -1, 1); // 좌표계를 윈도우 크기에 맞추기
}
void mousePressEvent(QMouseEvent* event) override {
currentLine.clear(); // 새로운 선을 시작할 때 초기화
currentLine.push_back(event->pos()); // 첫 번째 점 추가
update();
}
void mouseMoveEvent(QMouseEvent* event) override {
currentLine.push_back(event->pos()); // 마우스를 움직이는 동안 점 추가
update();
}
void mouseReleaseEvent(QMouseEvent* event) override {
currentLine.push_back(event->pos()); // 마지막 점 추가
lines.push_back({currentColor, currentLine}); // 현재 선과 색상을 저장
currentLine.clear(); // 새로운 선을 그리기 위해 초기화
update();
}
};
class MainWindow : public QWidget {
Scene* scene;
public:
MainWindow(QWidget* parent = nullptr) : QWidget(parent) {
scene = new Scene(this);
auto* layout = new QVBoxLayout;
auto* colorButton = new QPushButton("색상 변경", this);
connect(colorButton, &QPushButton::clicked, this, &MainWindow::changeColor);
layout->addWidget(colorButton);
layout->addWidget(scene);
setLayout(layout);
setWindowTitle("OpenGL Drawing with Mouse");
resize(800, 600);
}
private slots:
void changeColor() {
QColor color = QColorDialog::getColor(Qt::black, this, "색상 선택");
if (color.isValid()) {
scene->setColor(color);
}
}
};
int main(int argc, char** argv) {
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
색을 변경할 수 있게 UI도 하나 추가해보았다.
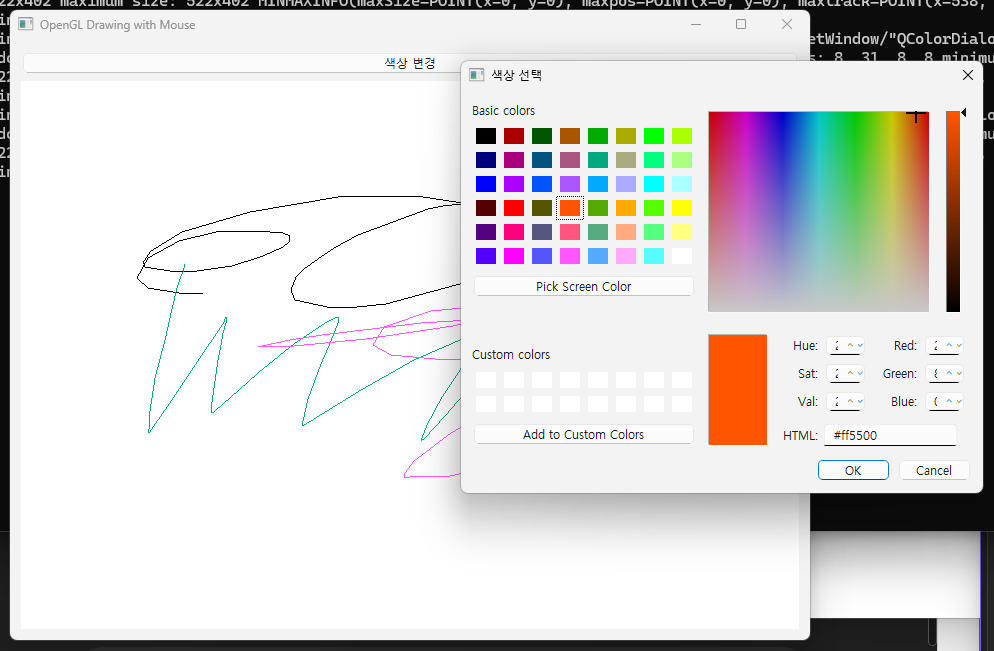
이렇게 간이 그림판 기능을 만들었다.
이전에 Q_OBJECT 관련된 moc 때문에 막혔었는데.
이번에는 해결하고 진행해보자.
728x90
반응형
'개발 · 컴퓨터공학' 카테고리의 다른 글
OmniIsaacGymEnvs 세팅하기 (5) | 2024.11.08 |
---|---|
Qt6 Opengl GLU 라이브러리 (6) | 2024.11.05 |
Qt 5 버전 오픈소스 실행하기 (7) | 2024.11.03 |
ubuntu 20.04 멀티 부팅 설치하기 (7) | 2024.10.31 |
ASE github 코드 환경 설정하기 - 1 [ASE: Large-Scale Reusable Adversarial Skill Embeddings for Physically Simulated Characters] (13) | 2024.10.30 |